Martin Doms' Design Study
Contents |
The problem
This design study is based on Trip, my COSC 325 team's project. The full system is a round-trip engineering tool that allows users to generate and modify UML diagrams from existing code, and push modifications through to the code. The program exists as a plugin for Eclipse written in Java.
The aspects of the project I am working on redesigning are the model and command infrastructure. The existing system is a transactional system, which means that the user makes arbitrary modifications to the UML representation, and at any time can commit those modifications to the code as a single unit of work.
There are two main problems with the existing system that I wish to solve. The first is in the model. The model is essentially a simplified representation of the code which is derived from a much more complex abstract syntax tree, provided by the Eclipse platform. The main problem with the current model is that due to poor design decisions early in the project (to be discussed later), modifications and extensions to the model are difficult. Many changes to the model result in bugs that would not exist in a more robust system.
The current model also breaks the single responsibility principle by including behaviour and data not related to modelling Java programs, such as location, size and status for diagram display purposes. The redesigned model is sharply focused on the problem of modelling a Java program.
The second issue is the command system. In the current system, UI actions result in the generation of Command objects which act on the model and the underlying code. For example, if the user renames a class on the UML diagram, the rename action afforded by the UI generates a rename Command object which is executed. There are no dependencies between the UI actions and the model, as the command infrastructure acts as an insulating layer between them. Commands can also be generated by events that occur on the file system, such as a file system listener creating a 'remove type' Command object when the user deletes a source file.
Because of the transactional nature of the program, actions that occur on the UI currently generate two commands: a command that changes the model (called a CommittableCommand) and the command to push that change into the code (called a CommitCommand). The CommittableCommand object is in charge of creating its own CommitCommand. This system worked well early in the project but as the program grew it has resulted in an explosion of CommittableCommand and CommitCommand subtypes which have become difficult to manage and often duplicate code.
This command system is completely thrown out and rewritten from scratch in a simpler and more elegant way.
The Redesign
The following are two UML diagrams showing the redesign of the system, followed by an explanation of them.
Model

The model UML diagram is necessarily complex, but is not difficult to understand. JavaComponent is the base class for all elements that are represented in a Java program or source file. JavaComponents can be composed of other JavaComponents (for example, JavaClasses are composed of JavaMethods, JavaFields and inner JavaClasses). Other elements are not composed of JavaComponents, such as JavaMethods, JavaParameters and JavaFields.
It could make semantic sense to say that JavaMethods are composed of JavaParameters, but I elected to keep methods as leaf nodes that contain parameters as lists because the order of parameters is important in a method declaration, and at the abstract level I defined child nodes in the composite as Collections, not Lists.
JavaRelationships are simple types that relate two JavaComponents. Each relationship has a source and target JavaComponent. In a UML class diagram these would be used for things like inheritance and dependency relationships, as shown in my UML diagram. They could also be used to model relationships between packages, messaging relationships and any kind of relationship where two Java elements are involved. Relationships in this manner are implicitly directed (ie, they have a source and a target) but clients are free to ignore this directionality.
Relationships between two types are resolved using strategies. As an example, if the client is interested in inheritance relationships from a given JavaType then they would instantiate an InheritanceRelationshipStrategy as that type's strategy and call the type's resolveRelationships() method. In a future design I would like to be able to compose multiple strategies, but time restrictions prevented this extension to the design.
In this model, relationships are generated and instantiated lazily as needed. Client code would be advised not to store collections of relationships, but allow JavaComponents to supply the relationships on demand. For example, asking a JavaClass for all of its source relationships would result in a collection containing relationships representing its superclass connection, implementation connections and association connections sourced from itself. This style of "stateless, pseduo-functional" programming can result in code that is easier to manage, more testable and less prone to state-related bugs.
Clients can therefore easily extend the system to allow for additional relationship types. For example if the client was modelling a UML Sequence Diagram, they would be free to extend JavaRelationship with a MessageRelationship subclass and generate their own strategy to resolve these relationships.
In this current version of the design I have stubbed out the JavaEnum class because the current version of Trip does not support Enums. I suspect that all that is required to extend the system to support enums is one more subclass of JavaMember but I have not yet investigated this fully.
Commands
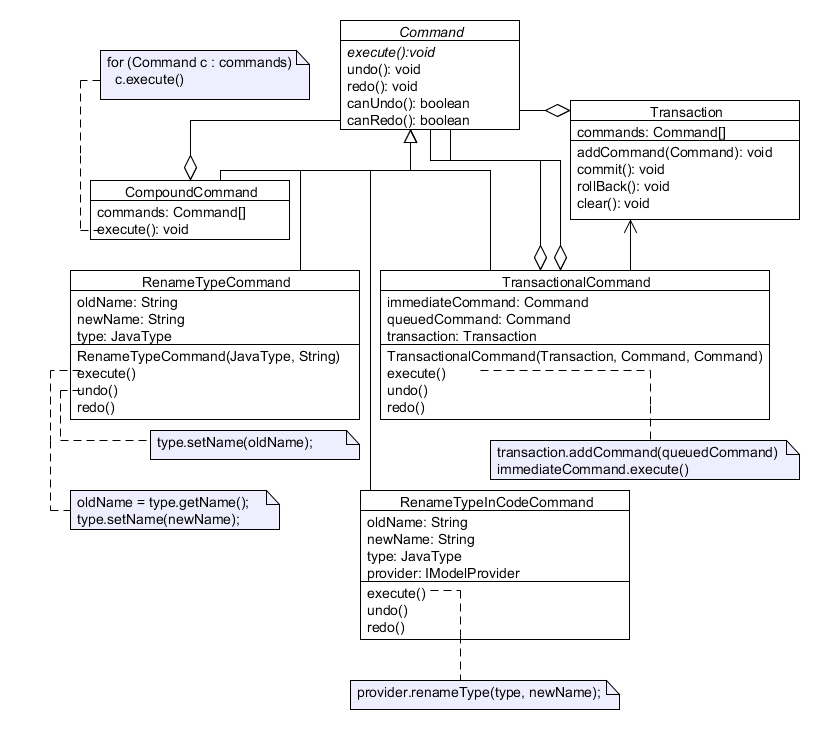
In the wider context of the project I am working on, interactions with the Model occur through commands. The benefits of this are
- Full separation of the UI from the model. Actions on the UI result in instantiation and execution of Command objects. Changes in the model can update the UI via the Observer pattern. This allows clients to choose from a number of different presentation patterns, including Presentation Model or MVC.
- The simplicity of implementation of undo and redo operations as clients can choose to implement a command stack or use an existing command stack in another framework.
- Commands can behave in a transactional manner, and commands can be composed into larger composite commands allowing for complex transactions composed from simple and easy to write commands.
In the Trip project for which this system is designed, some commands result in immediate changes in the model, while others require commands to be queued for later committing. An example of this is a Rename Class command. The class on the diagram that the user updates must be updated in the model immediately to reflect the change to the user, but the change is not pushed to code until the user commits this change.
To achieve this, we generate three commands in the action. The RenameTypeCommand renames the type in the model. A RenameTypeInCodeCommand renames the type in the code through a data layer (IModelProvider, not shown, as it is out of the scope of this design project). These two commands are added to a TransactionalCommand, which is a type of command which has an immediate and a queued component. When the TransactionalCommand is executed, it executes the immediate command, and queues the queued command in a transaction. Either of these commands could be a compound command, so a single transactional command could have multiple effects.
One of the biggest advantages to using the Command pattern is the ability to define actions on the model in a behaviour-centric (as opposed to data-centric) way. As an example, if the user issues an action to rename a class, the user interface programmer defines this behaviour as a RenameClassCommand, rather than calling a [modelObject].setName() method. This declarative style of programming can lead to fewer errors and abstracts any difficult behaviour involved with model actions into small, well-defined classes.
Note than on the diagram I have not shown any dependencies between the model and command objects. This was done to keep the diagrams understandable. Almost every command will have some dependency or containment relationship with one or more model objects, but each command will have different dependencies because they are object-specific. The model and commands are tightly coupled necessarily.
Patterns and Practices
The following design patterns can be found in this design:
Composite
The model implements composite pattern at the top level of abstraction. JavaComponents may be either leaf or composite components, with composite components containing a collection of JavaComponents. This is used because of the tree-like nature of a Java program, with packages nested inside of projects, types nest within packages etc. The reason I didn’t use regular containment (ie, packages containing a Set of types, etc.) is because of the complex nature of this tree – the rules around which items can be nested in which are complicated. For example, classes can be nested within classes, packages within packages and classes within packages, but not packages within classes.
We can control which components are allowed to be nested in which with business rules in the addChild method.
I have also used a modified version of Composite pattern in the command system. The CompoundCommand class can be thought of as a composite of commands and can even be composed of other CompositeCommands. In this case we have not used a particular leaf class, as all non-compound commands are assumed to be leafs.
Decorator
The decorator pattern is used to add behaviour and data to generic and array types in the model. For a complete description see the Model section above. This implementation of the decorator pattern is a classical one with nothing fancy going on. I could also have chosen to use Decorator for JavaParameterizedMethod in a similar way. I elected not to do this because I do not foresee other method decorators in this system and I wanted to Eliminate irrelevant classes. The UML class diagram above does not show the full implementation of Decorator (overridden methods are not shown). This has been done for simplicity in the diagram, and you may assume that the decorator has been implemented correctly.
Command
Quite obviously, the Command system implements the Command pattern .The advantages of this were described above, in the Command section. In this implementation the following items correspond to these Gang of Four labels
- Command: Command.
- ConcreteCommand: Any of the concrete subclasses of command.
- Client: The calling application.
- Invoker: Probably supplied by the client application. This could be either the same module as Client, or more often, a command stack of some kind to allow for undo and redo operations.
- Receiver: Model objects.
Observer
The model shown does not implement the full observer pattern, as clients are expected to supply the observers. However all model objects supply the standard Java Beans PropertyChangeSupport object to allow the Observer pattern to be used. In this case, model objects are Subjects in Gang of Four parlance, with JavaComponent being the abstract Subject and concrete implementations acting as ConcreteSubject classes.
Strategy
Strategies are used to resolve relationships between JavaComponents. This allows a flexible and extensible model that affords clients the ability to model any kind of relationship that can be inferred from the model presented, including relationships that can be inferred between components that are not explicitly modeled here (ie, if the client extends the model).
Other important maxims and heuristics
- Dependency inversion principle (violated) – This principle is slightly violated in this design. When children are added to concrete instances of JavaComponents, these concrete components must be aware of which kinds of concrete classes are allowable as children. For example, if the client tries to add a method as a direct child of a package, the system will throw an exception. Even though in most parts of the system we rely on abstractions as much as possible, in this case concrete classes must be aware of what other concrete classes are allowed.
- Liskov substitution principle (violated) – This principle is violated for a similar reason to the above. We can not necessarily substitute any concrete JavaComponent for any other when generating a valid Java syntax tree.
- Avoid concrete base classes – All base classes in this design are abstract. This allows maximum flexibility for any clients wanting to extend this model. The only exception to this rule is JavaParameterizedMethod which directly extends JavaMethod. In this case it did not seem useful to extend an abstract base JavaMethod with a concrete Method class which added no functionality (see Riel's Heuristic 3.7, Eliminate irrelevant classes).
- Open closed principle – This principle was important from the very beginning of this design. The reason is that a model like this could easily be expected to be extended later to allow more complex and more granular models. The model is easily extensible without modification. An example of this is the strategy pattern used for resolving relationships between components.
Further Criticisms and Ideas
It could be argued that the model objects in this design act only as "dumb data objects". They have no contact with the model provider objects and rely on third parties (the commands) for much of their behaviour. While this is a fair criticism, my defense is that the model was designed to be used anywhere that a simplified abstract representation of a Java program is required. Although the design is built for a UML diagramming tool, my goal was to make it generic enough that the entire Model package could be lifted into any kind of program, so I wanted to avoid dependencies on other parts of the system.
I also wanted to implement the visitor pattern to allow visitors to move through the model. However this design project is already pushing the boundaries of being too large for this assignment so I restrained myself in implementing this pattern. In a full implementation I would include an affordance for visitors, as this makes perfect sense for a design like this.
Implementation
Due to time constraints a full implementation of this system was not possible. However I was able to put together a fairly comprehensive suite of code to demonstrate both the model and command subsystems. Following are two UML diagrams generated from Trip using the code for this project. This code is all functioning, although it has not been thoroughly tested. The implementation is missing a lot of guard code and similar details and serves only as a proof of concept. The implementation also missing support for parameterized methods.
The Trip project is quite large with around 250 source files. Changing the model at this late stage in the project would be impossible, so this redesign did not make it into the actual product. However I hope to implement these changes in a similar project at some stage in the future.
Also note that the current version of Trip does not support self-relationships and has some issues with collections as association relationships, so not all relationships are shown perfectly on the following diagrams. However it is fairly close to an accurate representation of the source code.

The full source code (including Eclipse project and diagrams) can be downloaded here.